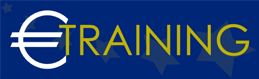

IT Management
Building Interactive Web Applications with C# and Blazor WebAssembly
Overview:
Introduction:
This training program focuses on equipping participants with the skills to develop dynamic and interactive web applications using C# and Blazor WebAssembly. It explores the fundamentals of Blazor WebAssembly, its integration with C#, and techniques to create responsive and modern web interfaces. Through it, participants will gain expertise in building scalable and efficient web applications aligned with industry best practices.
Program Objectives:
By the end of this program, participants will be able to:
-
Understand the core concepts of C# and its role in Blazor WebAssembly.
-
Develop and deploy interactive web applications using Blazor.
-
Implement robust UI/UX designs leveraging Blazor's component-based architecture.
-
Integrate backend services and manage data effectively.
-
Optimize performance and ensure scalability in Blazor applications.
Target Audience:
-
Web developers with basic knowledge of C#.
-
Software engineers looking to expand their skills in Blazor WebAssembly.
-
Developers aiming to create modern, interactive web applications.
-
IT professionals transitioning to full-stack development.
Program Outline:
Unit 1:
Introduction to C# and Blazor WebAssembly:
-
Overview of C# and its applications in web development.
-
Introduction to Blazor WebAssembly and its architecture.
-
Benefits of using Blazor over traditional JavaScript frameworks.
-
Setting up the development environment.
-
First steps: Creating and running a basic Blazor WebAssembly application.
Unit 2:
C# Fundamentals for Blazor Development:
-
Key features and syntax of C# relevant to Blazor.
-
Understanding classes, objects, and components.
-
Working with data types, collections, and LINQ in Blazor.
-
Exception handling and debugging in C#.
-
Writing reusable code for Blazor applications.
Unit 3:
Blazor Components and Razor Syntax:
-
Overview of Blazor components and their lifecycle.
-
Writing and organizing components using Razor syntax.
-
Passing parameters and managing state between components.
-
Leveraging templates and partial components.
-
Best practices for component reusability and modularity.
Unit 4:
Building Interactive User Interfaces:
-
Designing responsive and dynamic UI with Blazor.
-
Working with forms, input validation, and user feedback.
-
Implementing advanced UI elements such as grids, modals, and charts.
-
Using third-party libraries and integrating UI frameworks.
-
Customizing CSS and styling for a polished user experience.
Unit 5:
Data Management and Binding:
-
Understanding data binding in Blazor: one-way, two-way, and event binding.
-
Managing application state and context.
-
Connecting to APIs and consuming RESTful services.
-
Working with Entity Framework Core for database operations.
-
Handling asynchronous data updates and real-time data display.
Unit 6:
Routing and Navigation in Blazor Applications:
-
Configuring routing and navigation in Blazor WebAssembly.
-
Dynamic navigation between components and pages.
-
Using route parameters and query strings.
-
Implementing secure and conditional navigation.
-
Creating nested layouts and shared navigation menus.
Unit 7:
Authentication and Authorization:
-
Implementing authentication mechanisms in Blazor applications.
-
Working with ASP.NET Core Identity for user management.
-
Securing APIs and integrating OAuth and JWT.
-
Role-based and policy-based authorization in Blazor.
Unit 8:
Integrating Backend Services:
-
Overview of client-server architecture in Blazor WebAssembly.
-
Consuming APIs using HttpClient and GraphQL.
-
Handling data serialization and deserialization.
-
Managing server-side state with SignalR.
-
Real-time updates and push notifications in Blazor.
Unit 9:
Performance Optimization and Scalability:
-
Techniques for optimizing Blazor WebAssembly performance.
-
Lazy loading and optimizing component rendering.
-
Managing large datasets and reducing load times.
-
Deploying Blazor applications to scalable cloud platforms.
-
Monitoring and debugging performance issues.
Unit 10:
Testing and Deployment:
-
Writing unit tests for Blazor components and services.
-
Automating integration and end-to-end testing.
-
Packaging and deploying Blazor WebAssembly applications.
-
Managing version control and updates for live applications.
-
Strategies for maintaining and scaling applications post-deployment.